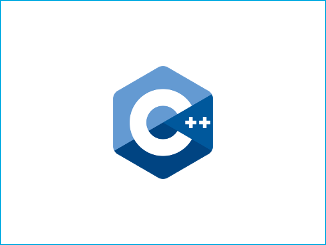
Get the length of std::array without instantiate an object.
Get the container length of std::array
You have declared an object with type std::array:
std::array<int, 3> data = {1,2,3};
To get the length of it is trivial:
auto length = data.size();
However, can you get the length of it just from the type? Imagine in your code base an array type is declared using an alias:
//in samples.h
using Samples = std::array<int, 3>;
Only the samples.h is visible to you and you want to declare another array with different element type but same size as Samples. You don’t want to hard-code another 3, cuz that reduces code reusability. You could do the following, but it is ugly:
Samples dummy{};
using FloatSamples = std::array<float, dummy.size()>;
Solution
Here I show a nicer way using some C++ template magic.
First we declare an template class which can take the std::array type as template argument:
//general case
template <typename Container>
struct helper
{
};
Then we add a specialization of the above template class. On the one hand, it let std::array type fall into this specialization category. On the other hand, we have an opportunity to extract the element type T and the array size N:
//specialization on array
template <typename T, size_t N>
struct helper<std::array<T, N>>
{
static constexpr std::size_t value{N};
};
If we put the above helper code into helper.h, and put everything together, then we have:
#include "samples.h"
#include "helper.h"
int main()
{
using FloatSamples = std::array<float, helper<Samples>::value>;
std::cout << helper<Samples>::value<< std::endl; // will print 3
return 0;
}
Summary
In the end, we are able to just use std::array type to induce the size of it at compile time, without a need to instantiate an instance of it. Thanks to the magic of template metaprogramming. The trick here we are using has a formal terminology, and is called meta function.
For more on meta function, I have a 10 min video explaining it in detail!
C++